Overview
In this lecture, we do a quick tour of C/C++'s features, starting from looking at interpretation vs compilation, and noting the language's various aspects (types, operators, flow control, etc.).
Interpretation vs compilation
Even before we begin looking at C++, let's consider the two common ways of "running code":
* interpretation
* compilation
Interpretation
* 'lightweight', casual, inviting, "fun"..
* code can be run piecemeal
* an interpreter (program) processes the code, executes it
* examples: JavaScript, Python, Perl, etc.
* an interactive interpreter is a 'REPL' (Read-Evaluate-Print-Loop) processor
Compilation
* "all at once": code can't be run piece by piece
* needs a compiler (program) to convert source code to an executable
* "serious", heavy..
* examples: C, C++, Java, FORTRAN..
* faster execution than interpreted code; provides the programmer, better control of memory usage
Interpreter: JavaScript
Here's a small example of using JavaScript. Examine the source for the page you loaded :)
As tiny as our program was (just one line!), it did not need to be compiled, it "just ran".
Interpreter: Python
Go to http://www.pythonfiddle.com, where there's a browser-based 'REPL' [Read-Evaluate-Print-Loop]. Type the following into the big textarea in the middle, then press the 'Run' button at the top left. Voila!
print "Hello, Python World!"
Again, our one-line program with Zen-like minimal syntax (no parantheses, no semi-colon) just directly ran, without compilation.
Hello World, in C++
Next, let us do a 'soup to nuts' running of a C++ program, to get a hang of the workflow.
We're going to use Code::Blocks, a very simple UI ["IDE"] to do C++. Please download it from this link - PC users should get codeblocks-20.03mingw-setup.exe, and Mac users, CodeBlocks-13.12-mac.zip. Unzip what you downloaded, now you're ready to start learning C++!!
Bring up Code::Blocks, create a new workspace (a container for a lot of 'project's), and within it (workspace), a new project (itself a container for a group of files that will together form a working program) called HelloWorld. Click on Workspace -> HelloWorld -> Sources -> main.cpp to bring it up in CodeBlock's text editor. Press F9, to watch the "source code get compiled, then executed".
Here is a (sped up!) clip that shows how to use Code::Blocks on a PC (the steps would be similar on a Mac), to compile and run a program/sourcecode:
In the source code editor panel, modify the 'cout' line that you see, to say:
cout << "Hello, C++ world!" << endl;
Having made the change, choose Build -> Build and run (F9 is a shortcut). After a few seconds, you'll see this:
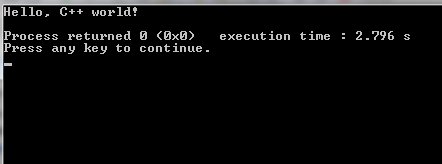
Nice! That was a successful compiling and running of our 'Hello World' program, from the source code file called main.cpp.
main.cpp is the source code file that contains the program. CodeBlocks provided both the file (main.cpp) and the code in it, as a convenience (to start us off). In general, we'd have an empty project, to which we'd add a blank file and type code into it, then compile and run.
If you look under the textarea, you'll see a smaller window that shows two compilation commands/steps:
mingw32-g++.exe -Wall -fexceptions -g -c
C:\Users\saty\Dropbox_RP\Dropbox\Public\!shared\courses\
CS455\m15\lectures\CB\HelloWorld\main.cpp -o obj\Debug\main.o
mingw32-g++.exe -o bin\Debug\HelloWorld.exe obj\Debug\main.o
When we say "compile and run", what we actually mean is compile, link and run - in other words, source code (our .cpp file) gets converted to a runnable (.exe), via a two step process:
* source file(s) -> compile -> object file(s) [.cpp => .o]
* object file(s) -> executable (*.o => .exe)
Putting them together:
source => compile => link => executable
IDEs run these commands on our behalf, by invoking an underlying compiler (mingw32-g++.exe in our case).
So, here's how to learn C++: write/modify code, press F9, write/modify code, press F9... repeat till you MASTER it :)
Hello World, in C++, 'by hand' [without an IDE]
It is instructive to put the IDE magic aside for a bit, and experience the joy of doing everything by hand - writing source code, compiling, linking, running.
To do so, we need to have a 'shell' (command line interface to an operating system) where we can issue our commands.
Let us do this on aludra/mizar, USC's ITS-provided Unix machines. We can use PuTTY or Bitvise (on a PC) to log on and launch a shell. Once we log in, we'll use a text editor (called emacs) to type in our Hello World code, and type in the compilation commands to turn our code into an executable (in an IDE, the exact same compilation steps are automatically done for us when we click on a button such as 'Build and Run'). It is always good to know what happens behind the curtain :)
Here is a screen-recording clip of me going through the steps:
Again: HelloWorld.cpp => HelloWorld.o => HelloWorld.exe
Our Hello World code
Let us briefly look at the various parts that make up the source (we'll examine these in more detail later):
#include <iostream>
using namespace std;
int main()
{
cout << "Hello, C++ world!" << endl;
return 0;
// Hello, CS455 :)
}// main()
Here are notes on the code, in no particular order:
* every C++ program MUST have a main function
* a function looks like aWord() { }
* #include brings in extra functionality
* iostream includes functionality (via cout) for printing to screen
* 'using' provides a way to avoid words over and over
* namespace std means that std is a 'wrapper' for functionality, in our case, cout and endl
* cout prints a bunch of supplied items to the screen (console)
* endl denotes a newline (carriage return) character
* return 0 means we're signalling success (to the OS, that invoked us)
* the lines inside the curly braces { and }, denote the 'body' of the main() function - execution of EVERY program starts at such a body (of main())
Syntax, semantics
Now it is time to examine things in a more structured way: we'll introduce language features, going from simple to complex. Pay attention to both the syntax (grammar, punctuation..) as well as semantics (meaning, intent). Let us look at these, in order [we will look at each in detail, during upcoming lectures]:
* variables, TYPEs
* operators
* expressions, statements
* flow control
* 'packaging': functions, classes, modules..
* other features (eg. file I/O, error handling etc)
Vars, types
Create a new CB (CodeBlocks) project called Basics_I, and use its main.cpp to try out all the code in the upcoming slides.
ALL languages have variables ("vars"), which are DATA HOLDERS: each variable has a name, and a TYPE (C++ is a 'strongly typed' language), and its purpose is to hold data that we'll write code will manipulate.
int i; // we're creating an int variable named 'i'
cout << i << endl; // what will i be?
Was 'i', what you expected it to be? To be sure, always remember to INITIALIZE vars right when you create them.
int i=0;
cout << i << endl;
int stands for 'integer', a built-in C++ DATATYPE. Likewise, float is the floating point type, double is double precision floating point, and long is a long integer.
int i=1024;
float wheelRadius=6.54; // note naming convention
double angle = 0.55729;
long particleId = 123456789;
cout << i << " " << wheelRadius << " ";
cout << angle << " " << particleId << endl;
Each datatype has a specific amount (number) of bytes of memory allocated to it (for holding data). A command called sizeof() provides a datatype's size.
cout << "Data type Bytes" << endl;
cout << "char: " << sizeof(char) << endl;
cout << "short: " << sizeof(short) << endl;
cout << "int: " << sizeof(int) << endl;
cout << "long: " << sizeof(long) << endl;
cout << "float: " << sizeof(float) << endl;
cout << "double: " << sizeof(double) << endl;
cout << "long double: " << sizeof(long double) << endl;
Note that sizeof() specifies size, in bytes, a byte being 8 bits. For example, sizeof(int) is 4 bytes, which is 32 bits.
Here is more, on types.
Operators
Operators (+, * etc) help perform numerical calculations, and also comparisons, etc.
double x = (1*1 + 2*2 + 3*3)/(4*4);
int x=4, y=6;
// does x equal y? If yes, areTheyEqual will be assigned 1; if not, 0
int areTheyEqual = (x==y);
cout << areTheyEqual << endl;
C++ has a big list of operators! The 'bitwise' operators are less commonly used, so you can skip them for now.
Expressions, statements
Expressions (informally, "formulae") occur on the RIGHT side of an = sign.. When a variable occurs in an expression, its corresponding value is used in the calculation. Eg:
x + y
(x*y)/z
degCelcius*9.0/5 + 32
z
24
A statement (informally, a 'sentence') is of the form 'variable = expression' - in other words, it includes a variable assignment as well. Also, statements need to end with a semi-colon.
degFahrenheit = degCelcius*9.0/5 + 32;
r = 255-r;
Flow control [loops, branches]!
So far, we've seen statements that lead to SEQUENTIAL execution. What if we need to loop (repeat) a bunch of code, or need to branch (execute one block of code versus another)??
In C++, we use for() and while() to loop, and if() and switch() to branch. These are called flow control constructs, because they help control the flow of execution of code (what runs after what).
Looping and branching can be combined in myriad ways, to express program logic (what you want your code to do). So be sure to understand the constructs very, very well!